Session 3, #4: Parsing USGS Data
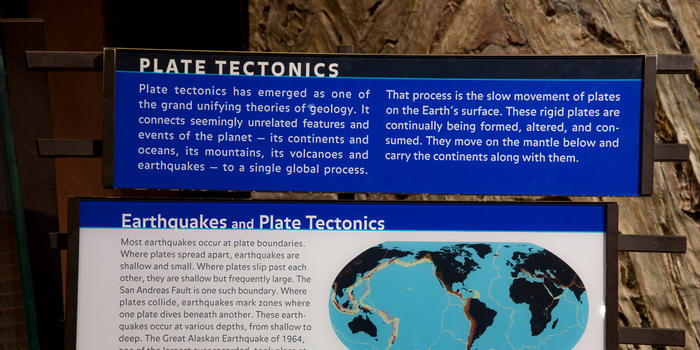
Python has some built-in commands for breaking down complicated lines like those in the CSV file:
time,latitude,longitude,depth,mag,magType,nst,gap,dmin,rms,net,id,updated,place,type,horizontalError,depthError,magError,magNst,status,locationSource,magSource 2017-01-17T11:48:48.530Z,5.4319,94.6079,54.55,5.6,mb,,67,2.338,1,us,us10007tps,2017-01-17T12:14:46.732Z,"80km W of Banda Aceh, Indonesia",earthquake,7.4,5.8,0.045,173,reviewed,us,us(each line is so long, it goes off the edge of the window...)
Let's focus in on the second line:
obsData = '2017-01-17T11:48:48.530Z,5.4319,94.6079,54.55,5.6,mb,,67,2.338,1,us,us10007tps,2017-01-17T12:14:46.732Z,"80km W of Banda Aceh, Indonesia",earthquake,7.4,5.8,0.045,173,reviewed,us,us'We will give this data about the observed earthquake a name, obsData, to make it easier to refer and use. In Python, named items are called variables. All of our turtles (teddy, tommy, and friends) are variables in our programs.
Our goal is plot thousands of earthquake observerations, so, it would be helpful to automatically extract the longitude and latitude from each line. Here's the steps we need:
- Split up a line into the columns, and
- Convert those columns (which are stored as words) to numbers
- Split up a line into the columns:
We use split() to split up the line, breaking on commas:
columns = obsData.split(",")
- Convert those columns (which are stored as words) to numbers:
We then take the column after the first comma (column[1]) and convert it from words to a decimal. We do the same for the second column:
float(columns[1]) float(columns[2])We then store in variables, lat and long.
lat = float(columns[1]) lon = float(columns[2])
We can then use the numbers stored in the variables lon and lat to mark the longitude and latitude from the file to the world map:
Challenge: At the bottom of the program, there are two variables, obsData2 and obsData3, that contain the next two lines of USGS data. Stamp the points stored in obsData2 and obsData3 on the map.